
1、学生类的代码如下
public class Student implements Comparable<Student> { private String name; private int chinese; private int math; private int english; public Student() { } public Student(String name, int chinese, int math, int english) { this.name = name; this.chinese = chinese; this.math = math; this.english = english; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getChinese() { return chinese; } public void setChinese(int chinese) { this.chinese = chinese; } public int getMath() { return math; } public void setMath(int math) { this.math = math; } public int getEnglish() { return english; } public void setEnglish(int english) { this.english = english; } @Override public String toString() { return "Student{" + "name='" + name + '\'' + ", chinese=" + chinese + ", math=" + math + ", english=" + english + '}'; } public int getSum() { return chinese + math + english; } @Override public int compareTo(Student o) { //按总分就行排序 int result = this.getSum() - o.getSum(); //次要条件 //如果总分成绩再相同,按语文成绩排序 result = result == 0 ? this.getChinese() - o.getChinese() : result; //如果语文成绩再相同,按数学成绩排序 result = result == 0 ? this.getMath() - o.getMath() : result; //如果数学成绩再相同,按英语成绩排序 result = result == 0 ? this.getEnglish() - o.getEnglish() : result; //如果英语成绩再相同,根据姓名排序 result = result == 0 ? this.getName().compareTo(o.getName()): result; return result; } }
2-5、步骤代码如下
public class MyCollection6 { public static void main(String[] args) { TreeSet<Student> st = new TreeSet<>(); Student s1 = new Student("dahei", 80, 80, 80); Student s2 = new Student("erhei", 90, 90, 90); Student s3 = new Student("xiaohei", 100, 100, 100); st.add(s1); st.add(s2); st.add(s3); for (Student t : st) { System.out.println(t); } } }
在此次set的底层原理
它在底层会把这三个对象封装成三个节点,如下图形式
1、在添加节点240时,它会破会红黑规则,原因:根节点必须是黑色。所以要把节点240变为黑色,样式如下图
2、添加节点270的结果如下图
3、添加节点300后,它破坏红黑规则。原因:不能出现两个红色节点相连
解决过程如下
解决依据
其父节点为红色,叔叔节点是黑色
1.将“父节点270”设为“黑色”
2. 将“祖父节点240”设为“红色”
3.以祖父节点为支点进行旋转(是左旋还是右旋要看左右俩边的大小,即左右俩边层数的比较)
结果如下图
评价
排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

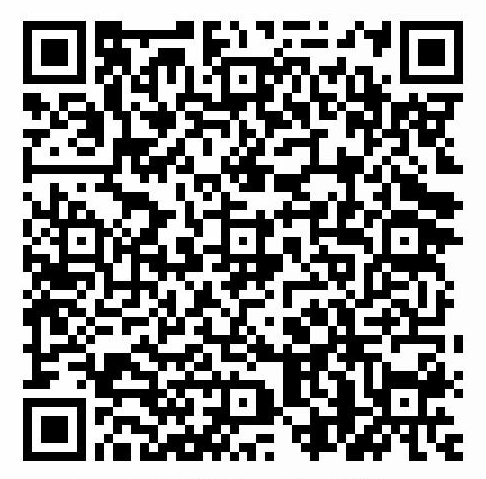
欢迎加群交流技术