
一、
二、
案例、
环境、
1、pom.xml的坐标
<!-- servlet规范--> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency> <!-- jsp --> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.1</version> <scope>provided</scope> </dependency> <!-- spring--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>5.1.9.RELEASE</version> </dependency> <!-- spring Mvc--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.1.9.RELEASE</version> </dependency> <!-- spring Web--> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>5.1.9.RELEASE</version> </dependency> <!--json的3个坐标--> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.9.0</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.9.0</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.9.0</version> </dependency>
2、spring-mvc.xml的配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd"> <!-- 扫描sprig的bean--> <context:component-scan base-package="com.itheima"/> <!-- mvc驱动--> <mvc:annotation-driven/> <!-- 放行静态资源--> <mvc:resources mapping="/js/**" location="/js/"/> </beans>
3、web.xml的配置
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <!--用于解决中文乱码--> <filter> <filter-name>CharacterEncodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>CharacterEncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!--拦截--> <servlet> <servlet-name>DispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath*:spring-mvc.xml</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>DispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
4、ajax.jsp即Ajax的用法所在位置,注意:在用Ajax前要下载jQuery
<%@page pageEncoding="UTF-8" language="java" contentType="text/html;UTF-8" %> <a href="javascript:void(0) ; " id="testAjax">访问springmvc后台controller</a><br/> <a href="javascript:void(0) ;" id="testAjaxPojo">访问springmvc后台controller,传递Json格式PoJo</a><br/> <a href="javascript:void(0);" id="testAjaxList">访问springmvc后台controller,传递Json格式List</a><br/> <a href="javascript :void(0); " id="testCross">跨域访问</a><br/> <%--本地下载的jquery位置--%> <script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery-3.3.1.main.js"></script> <script type="text/javascript"> $(function () { // 为id="testAjax"的组件绑定点击事件 $("#testAjax").click(function () { //发送异步调用 $.ajax({ //请求方式: type: "POST", //请求的地址 url: "ajaxController", //请求参数(也就是请求内容) data: "ajax message", //响应正文类型 dataType: "text", //请求正文的MIME类型 contentType: "application/text" }); }); //为id="testAjaxPojo"的组件绑定点击事件 $("#testAjaxPojo").click(function () { $.ajax({ type: "POST", url: "ajaxPojoToController", data: '{"name":"Jock","age":39}', dataType: "text", contentType: "application/json", }); }); //为id="testAjaxList"的组件绑定点击事件 $("#testAjaxList").click(function () { $.ajax({ type: "POST", url: "ajaxListToController", data: '[{"name":"Jock","age":39},{"name":"Jock2","age":33}]', dataType: "text", contentType: "application/json", }); }); //为id="testAjaxReturnString"的组件绑定点击事件 $("#testAjaxReturnString").click(function () { //发送异步调用 $.ajax({ type: "POST", url: "ajaxReturnString", //回调函数 success: function (data) { //打印返回结果 alert(data); } }); }); }) </script>
5、
6、测试
6.1、访问ajax.jsp的网页
6.2、响应结果
评价
排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

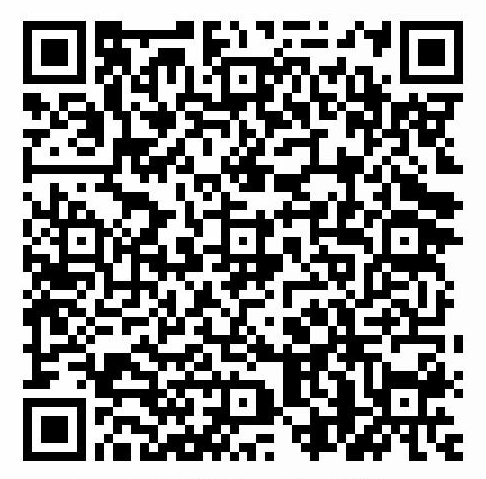
欢迎加群交流技术