
/// <summary> /// JSApi 调用类 /// </summary> public class WxPayJsApi { //获取 调起支付 API参数 public string GetJsApiParameters(string prepay_id) { WcPayChooseRequest request = new WcPayChooseRequest( WxPayConfig.APPID, WxPayUitls.GenerateTimeStampString(), WxPayUitls.GenerateNonceStr(), "prepay_id=" + prepay_id, "RSA"); request.paySign = WxPayEncrypt.MakePaySign(request.GetMessage(request)); string parameters = request.ToJsonStr(); return parameters; } /// <summary> /// 返回 config 注入参数 /// </summary> /// <param name="url"></param> /// <returns></returns> public string GetJsApiConfig(string url) { WxPayApiJSDK config = new WxPayApiJSDK(true, WxPayConfig.APPID, WxPayUitls.GenerateTimeStampString(), WxPayUitls.GenerateNonceStr(), url ); //正式上线 需要添加正确api列表 var enumValues = Enum.GetValues(typeof(WechatPayApiEnum)); foreach (Enum value in enumValues) { config.JsApiList.Add(value.ToString()); } string parameters = config.ToJsonToFrontStr(); return parameters; } } /// <summary> /// 注入 JSDK config 类 /// </summary> public class WxPayApiJSDK { private bool debug; private string appId; private string timestamp; private string nonceStr; private string signature; private List<string> jsApiList = new List<string>(); private string url; private string tokenUrl = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={0}&secret={1}"; public WxPayApiJSDK(bool debug, string appId, string timestamp, string nonceStr, string url) { this.debug = debug; this.appId = appId; this.timestamp = timestamp; this.nonceStr = nonceStr; this.url = url; this.signature = GetConfigSignature(); } /// <summary> /// 是否开启调试模式 /// </summary> public bool Debug { get { return debug; } set { debug = value; } } /// <summary> /// 必填,公众号的唯一标识 /// </summary> public string AppId { get { return appId; } set { appId = value; } } /// <summary> /// 必填,生成签名的时间戳 /// </summary> public string Timestamp { get { return timestamp; } set { timestamp = value; } } /// <summary> /// 必填,生成签名的随机串 /// </summary> public string NonceStr { get { return nonceStr; } set { nonceStr = value; } } /// <summary> /// 必填,签名 /// </summary> public string Signature { get { return signature; } set { signature = value; } } /// <summary> /// 必填,需要使用的JS接口列表 /// </summary> public List<string> JsApiList { get { return jsApiList; } set { jsApiList = value; } } public string Url { get { return url; } set { url = value; } } /// <summary> /// 生成注入config签名 /// </summary> /// <param name="request"></param> /// <returns></returns> private string GetConfigSignature() { Hashtable hs = new Hashtable(); hs.Add("jsapi_ticket", Getjsapi_ticket());//获取的 hs.Add("noncestr", NonceStr); hs.Add("timestamp", Timestamp); hs.Add("url", Url); //得到string1 string string1 = formatParameters(hs); //对string1进行sha1签名 Signature = GetHashSigntrue(string1); return Signature; } /// <summary> /// 参数名ASCII码从小到大排序(字典序) /// </summary> /// <param name="parameters"></param> /// <returns></returns> private string formatParameters(Hashtable parameters) { StringBuilder sb = new StringBuilder(); ArrayList akeys = new ArrayList(parameters.Keys); akeys.Sort(); foreach (string k in akeys) { string v = (string)parameters[k];//防止参数不是字符串 sb.Append(k.ToLower() + "=" + v + "&"); } //去掉最后一个& if (sb.Length > 0) { sb.Remove(sb.Length - 1, 1); } return sb.ToString(); } /// <summary> /// 进行sha1签名 /// </summary> /// <param name="str"></param> /// <returns></returns> public static string HashCode(string str) { string rethash = ""; try { SHA1 hash = SHA1.Create(); rethash = Convert.ToBase64String(hash.ComputeHash(Encoding.UTF8.GetBytes(str))); } catch (Exception ex) { string strerr = "Error in HashCode : " + ex.Message; } return rethash; } public string GetHashSigntrue(string str) { var sha1 = new SHA1Managed(); var sha1bytes = System.Text.Encoding.GetEncoding("UTF-8").GetBytes(str); byte[] resultHash = sha1.ComputeHash(sha1bytes); string sha1String = BitConverter.ToString(resultHash).ToLower(); sha1String = sha1String.Replace("-", ""); return sha1String; } public string GetAccess_Token() { var cache = CacheHelper.Instance; string access_token = string.Empty; if (cache.GetValue(WxPayCacheKeys.JSAPI_TICKET) == null) { string AppID = WxPayConfig.APPID; string AppSecret = WxPayConfig.APPSECRET; string url = string.Format(tokenUrl, AppID, AppSecret); System.Net.WebRequest wrq = System.Net.WebRequest.Create(url); wrq.Method = "GET"; System.Net.WebResponse wrp = wrq.GetResponse(); System.IO.StreamReader sr = new System.IO.StreamReader(wrp.GetResponseStream(), System.Text.Encoding.GetEncoding("UTF-8")); string strResult = sr.ReadToEnd(); JObject pairs = JObject.Parse(strResult); access_token = pairs["access_token"].ToString(); } else access_token = cache.GetValue(WxPayCacheKeys.ACCESS_TOKEN).ToString(); return access_token; } /// <summary> /// 签名所需要的凭证 /// </summary> /// <returns></returns> public string Getjsapi_ticket() { var cache = CacheHelper.Instance; string Jsapi_ticket = string.Empty; string access_token = string.Empty; if (cache.GetValue(WxPayCacheKeys.JSAPI_TICKET) == null) { string url = string.Empty; string strResult = string.Empty; System.Net.WebRequest wrq = null; System.Net.WebResponse wrp = null; System.IO.StreamReader sr = null; JObject pairs = null; if (cache.GetValue(WxPayCacheKeys.ACCESS_TOKEN) == null) { string AppID = WxPayConfig.APPID; string AppSecret = WxPayConfig.APPSECRET; url = string.Format("https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid={0}&secret={1}", AppID, AppSecret); wrq = System.Net.WebRequest.Create(url); wrq.Method = "GET"; wrp = wrq.GetResponse(); sr = new System.IO.StreamReader(wrp.GetResponseStream(), System.Text.Encoding.GetEncoding("UTF-8")); strResult = sr.ReadToEnd(); pairs = JObject.Parse(strResult); if (!strResult.Contains("access_token")) { return "not in whitelist rid"; } access_token = pairs["access_token"].ToString(); sr.Close(); } else { access_token = cache.GetValue(WxPayCacheKeys.ACCESS_TOKEN).ToString(); } url = string.Format("https://api.weixin.qq.com/cgi-bin/ticket/getticket?access_token={0}&type=jsapi", access_token); wrq = System.Net.WebRequest.Create(url); wrp = wrq.GetResponse(); sr = new System.IO.StreamReader(wrp.GetResponseStream(), System.Text.Encoding.GetEncoding("UTF-8")); string backStr = sr.ReadToEnd(); pairs = JObject.Parse(backStr); var code = pairs["errcode"].ToString(); if (code != "0") { return pairs["errmsg"].ToString(); } Jsapi_ticket = pairs["ticket"].ToString(); cache.InsertAndAbsoluteExp(WxPayCacheKeys.ACCESS_TOKEN, access_token, WxPayCacheKeys.ExpirationTime);//写入缓存 cache.InsertAndAbsoluteExp(WxPayCacheKeys.JSAPI_TICKET, Jsapi_ticket, WxPayCacheKeys.ExpirationTime);//写入缓存 } else Jsapi_ticket = cache.GetValue(WxPayCacheKeys.JSAPI_TICKET).ToString(); return Jsapi_ticket; } }
评价
排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2024TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

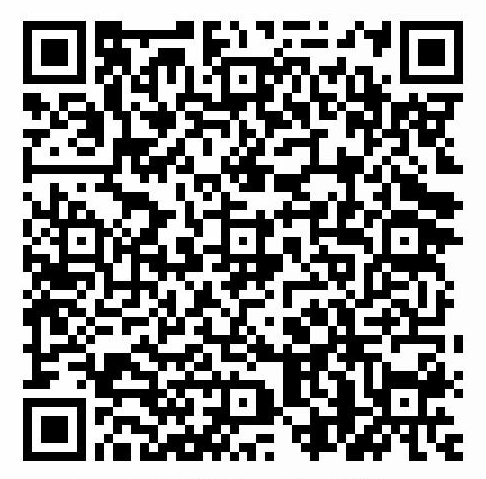
欢迎加群交流技术