
全称:【天眼数聚】人像实人认证-人像比对-人脸身份证比对-人脸三要素对比-人证比对-人脸身份证实名认证-人脸对比实名认证-人脸认证
json序列化所需的Dto,注意:Dto属性名称一定要和json数据名称一致
json格式:
{
"success": false,
"code": 400, //指返回码,并非http状态码 "msg": "入参错误,身份证号[idcard]格式错误",
"data": { }
}
/// <summary> /// 返回身份证官方信息Dto /// </summary> public class ResultDto { /// <summary> /// 信息 /// </summary> public string Msg { get; set; } /// <summary> /// 成功 /// </summary> public string Success { get; set; } /// <summary> /// 代码 /// </summary> public string Code { get; set; } /// <summary> /// 子集数据 /// </summary> public ResultDto2 Data { get; set; } } /// <summary> /// 返回子集身份证官方信息Dto /// </summary> public class ResultDto2 { /// <summary> /// 订单号 /// </summary> public string Order_no { get; set; } /// <summary> /// score 比较结果分值,0-1之间的小数,参考指标:0.40以下系统判断为不同人; /// 0.40-0.44不能确定是否为同一人;0.45及以上系统判断为同一人 /// </summary> public string Score { get; set; } /// <summary> /// 比较结果的描述 /// </summary> public string Msg { get; set; } /// <summary> /// 比较结果返回码,见incorrect详解 /// </summary> public string Incorrect { get; set; } /// <summary> /// 性别 /// </summary> public string Sex { get; set; } /// <summary> /// 生日 /// </summary> public string Birthday { get; set; } /// <summary> /// 地址 /// </summary> public string Address { get; set; } }
//Dto结构
所需的依赖包
using Abp.UI; using Newtonsoft.Json; using Newtonsoft.Json.Linq; using System; using System.IO; using System.Net; using System.Net.Security; using System.Security.Cryptography.X509Certificates; using System.Text; using ZZY.Finance.FaceIDcards.Dto;
具体代码
namespace ZZY.Finance.FaceIDcards { /// <summary> /// 人脸身份证对比应用层 /// </summary> public class FaceIDcard : FinanceAppServiceBase, IFaceIDcard { /// <summary> /// 依赖注入 /// </summary> public FaceIDcard() { } private const String host = "https://faceidcardb.shumaidata.com"; private const String path = "/getfaceidb"; private const String method = "POST"; //appcode :个人的appcode private const String appcode = "1233211234567"; /// <summary> /// 人脸身份证对比查询 /// </summary> /// <returns></returns> public ResultDto GetFaceIDcardJsonToDto(FaceIDcardQueryDto Query) { try { String url = host + path; string body = ""; body += "idcard=" + Query.IDNo; body += "&name=" + Query.Name; body += "&url=" + Query.Url; var Result = GetFunction(url, body); return JsonStringToObj<ResultDto>(Result); } catch { throw new UserFriendlyException("系统提示:官方身份证信息获取失败!"); } } /// <summary> /// sjon反序列化 /// </summary> /// <typeparam name="ObjType"></typeparam> /// <param name="JsonString"></param> /// <returns></returns> protected static ObjType JsonStringToObj<ObjType>(string JsonString) where ObjType : class { return JsonConvert.DeserializeObject<ObjType>(JsonString); } /// <summary> /// 调用接口-POST方法 /// </summary> /// <param name="url"></param> /// <param name="bodys"></param> /// <returns></returns> protected static string GetFunction(string url, string bodys) { String querys = ""; HttpWebRequest httpRequest = null; if (0 < querys.Length) { url = url + "?" + querys; } if (host.Contains("https://")) { ServicePointManager.ServerCertificateValidationCallback = new RemoteCertificateValidationCallback(CheckValidationResult); httpRequest = (HttpWebRequest)WebRequest.CreateDefault(new Uri(url)); } else { httpRequest = (HttpWebRequest)WebRequest.Create(url); } httpRequest.Method = method; httpRequest.Headers.Add("Authorization", "APPCODE " + appcode); //根据API的要求,定义相对应的Content-Type httpRequest.ContentType = "application/x-www-form-urlencoded; charset=UTF-8"; if (0 < bodys.Length) { byte[] data = Encoding.UTF8.GetBytes(bodys); using (Stream stream = httpRequest.GetRequestStream()) { stream.Write(data, 0, data.Length); } } HttpWebResponse response = (HttpWebResponse)httpRequest.GetResponse(); Stream myResponseStream = response.GetResponseStream(); StreamReader myStreamReader = new StreamReader(myResponseStream, Encoding.UTF8); string retString = myStreamReader.ReadToEnd(); return retString; } /// <summary> /// 忽略证书认证错误处理的函数 /// </summary> /// <param name="sender"></param> /// <param name="certificate"></param> /// <param name="chain"></param> /// <param name="errors"></param> /// <returns></returns> public static bool CheckValidationResult(object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors errors) { return true; } } }
评价
排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2024TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

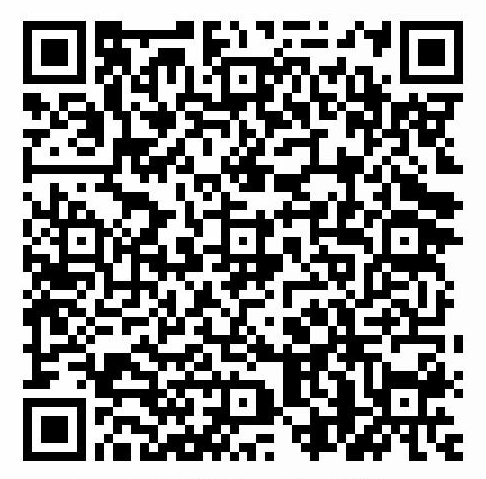
欢迎加群交流技术