
我们需要展示一个这样的表格数据
接口返回的数据源是这个样子
有评估,实验,产教的数据接口回来,这里只展示了评估的数据接口作为示例,相当于每个平台每月一条数据:
{
"code": 0,
"data": [
{
"systemName": null,
"year": 2024,
"month": 9,
"quantity": 0,
"completPr": 0.2164,
"planCompletePr": 0.8455172413793103448275862069
},
{
"systemName": null,
"year": 2024,
"month": 10,
"quantity": 0,
"completPr": 0.3224,
"planCompletePr": 0.4787902592301649646504320503
},
{
"systemName": null,
"year": 2024,
"month": 11,
"quantity": 0,
"completPr": 0.0,
"planCompletePr": 0.0
},
{
"systemName": null,
"year": 2024,
"month": 12,
"quantity": 0,
"completPr": 0.0,
"planCompletePr": 0.0
},
{
"systemName": null,
"year": 2025,
"month": 1,
"quantity": 0,
"completPr": 0.0,
"planCompletePr": 0.0
}
],
"success": true,
}
接口出来的数据源要先处理成这个样子的,这样行专列好处理一点
const rawData = [
{ "type": "评估", "month": 9, "CompleteType": "目标达成率", "CompleteValue": 0.2164 },
{ "type": "评估", "month": 10, "CompleteType": "整体完成率", "CompleteValue": 0.7164 },
{ "type": "审计", "month": 9, "CompleteType": "目标达成率", "CompleteValue": 0.6164 },
{ "type": "审计", "month": 10, "CompleteType": "整体完成率", "CompleteValue": 0.5164 },
];
具体的处理逻辑
数据源按照类型(type
)、月份(month
)、完成类型(CompleteType
)和完成值(CompleteValue
)组织好的,那么处理起来会简单很多。你可以直接使用这个数据来生成表格,其中月份可以转换为“9月”、“10月”等格式,完成值可以格式化为百分比。
以下是一个处理这种数据源的JavaScript函数,它将数据转换为适合Element UI表格的格式:
const rawData = [
{ "type": "评估", "month": 9, "CompleteType": "目标达成率", "CompleteValue": 0.2164 },
{ "type": "评估", "month": 10, "CompleteType": "整体完成率", "CompleteValue": 0.7164 },
{ "type": "审计", "month": 9, "CompleteType": "目标达成率", "CompleteValue": 0.6164 },
{ "type": "审计", "month": 10, "CompleteType": "整体完成率", "CompleteValue": 0.5164 },
// 可以添加更多数据
];
const monthMap = {
9: "9月",
10: "10月",
// 添加更多月份映射
};
const transformData = (data) => {
const result = [];
const types = [...new Set(data.map(item => item.type))];
const completeTypes = [...new Set(data.map(item => item.CompleteType))];
types.forEach(type => {
completeTypes.forEach(completeType => {
const row = {
type: type,
indicator: completeType,
...Object.keys(monthMap).reduce((acc, month) => {
const value = data.find(item => item.type === type && item.CompleteType === completeType && item.month === parseInt(month, 10))?.CompleteValue;
let returnValue = "0%"
if(value>=0){
returnValue=(value * 100).toFixed(2)
}
else{
returnValue="-"
}
return { ...acc,[monthMap[month]]:returnValue };
// return { ...acc, [monthMap[month]]: value ? (value * 100).toFixed(2) + "%" : "-" };
}, {})
};
result.push(row);
});
});
return result;
};
const tableData = transformData(rawData);
console.log(tableData);
这个函数transformData
会生成一个数组,每个元素都是一个对象,包含type
(类型)、indicator
(指标,即CompleteType
)、以及各个月份的完成值(已经转换为百分比格式)。如果某个月份没有对应的数据,则显示为'-'
。
在Vue组件中,你可以直接使用这个tableData
来渲染Element UI表格:
<template>
<el-table :data="tableData">
<el-table-column prop="type" label="类型" />
<el-table-column prop="indicator" label="指标" />
<el-table-column
v-for="(month, key) in monthMapValues"
:key="key"
:label="month"
:prop="month"
:formatter="formatPercentage"
/>
</el-table>
</template>
<script>
export default {
data() {
return {
tableData: [], // 这里将使用transformData函数的结果来赋值
monthMapValues: [
"9月",
"10月",
// 添加更多月份
]
};
},
created() {
this.tableData = transformData(this.rawData); // 假设this.rawData是上面定义的rawData
},
methods: {
formatPercentage(row, column, cellValue) {
// 这个方法用于格式化百分比,但在这个例子中我们已经在transformData中完成了格式化
// 所以这里可以直接返回cellValue,或者根据需要进行进一步处理
return cellValue;
},
// 如果你想在组件中直接使用transformData函数,也可以将它定义在这里
// 然后调用this.transformData(this.rawData)来赋值给this.tableData
// 但注意,这样做的话,你需要将rawData也定义在data中,或者以props的形式传入组件
},
// 注意:由于我们在created钩子中已经赋值了tableData,所以不需要再使用computed属性来动态计算它
// 同时,我们直接在data中定义了monthMapValues数组来作为表格的列名(月份)
};
</script>
<!-- 注意:在实际代码中,你应该将rawData替换为从API或其他来源获取的数据 -->
<!-- 并且确保在created钩子或其他适当的生命周期钩子中调用transformData函数 -->
请注意,上面的Vue组件示例中,我假设rawData
已经在组件的某个地方定义好了(可能是作为data属性,或者是作为props传入的)。在实际应用中,你需要根据你的数据来源来相应地调整这部分代码。同时,由于我们已经在transformData
函数中完成了百分比的格式化,所以formatPercentage
方法在这里可能并不需要做什么额外的处理,除非你有其他的格式化需求。
更多详细逻辑参考
欢迎加群讨论技术,1群:677373950(满了,可以加,但通过不了),2群:656732739。有需要软件开发,或者学习软件技术的朋友可以和我联系~(Q:815170684)
评价
排名
9
文章
115
粉丝
5
评论
5
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

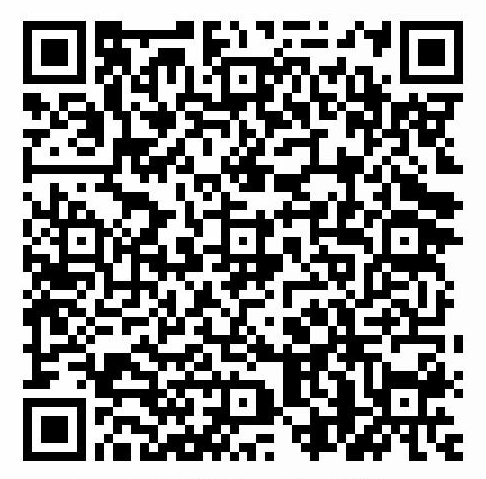
欢迎加群交流技术