
Windows下安装RabbitMQ:http://www.tnblog.net/aojiancc2/article/details/232
消息队列rabbitmq介绍:http://www.tnblog.net/aojiancc2/article/details/2332
(以上引用自大佬文章)
打开idea,创建springboot项目
依赖如下:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency>
yml文件
创建conf目录,创建交换机的配置类ExchangeConfig
package com.cy.demo.rabbitmq.conf; import org.springframework.amqp.core.DirectExchange; import org.springframework.amqp.core.FanoutExchange; import org.springframework.amqp.core.TopicExchange; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class ExchangeConfig { /** * 1.定义direct exchange,绑定queueTest * 2.durable="true" rabbitmq重启的时候不需要创建新的交换机 * 3.direct交换器相对来说比较简单,匹配规则为:如果路由键匹配,消息就被投送到相关的队列 * fanout交换器中没有路由键的概念,他会把消息发送到所有绑定在此交换器上面的队列中。 * topic交换器你采用模糊匹配路由键的原则进行转发消息到队列中 * key: queue在该direct-exchange中的key值,当消息发送给direct-exchange中指定key为设置值时, * 消息将会转发给queue参数指定的消息队列 */ //direct交换器 @Bean public DirectExchange directExchange(){ DirectExchange directExchange = new DirectExchange(RabbitMqConfig.EXCHANGE,true,false); return directExchange; } /*//fanout交换器 @Bean public FanoutExchange fanoutExchange(){ FanoutExchange fanoutExchange = new FanoutExchange(RabbitMqConfig.EXCHANGE,true,false); return fanoutExchnge; }*/ /* //topic交换器 @Bean public TopicExchange topicExchange(){ TopicExchange topicExchange = new TopicExchange(RabbitMqConfig.EXCHANGE,true,false); return topicExchange; }*/ }
创建QueueConfig配置类,配置队列
package com.cy.demo.rabbitmq.conf; import org.springframework.amqp.core.Queue; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class QueueConfig { @Bean public Queue firstQueue() { /** durable="true" 持久化 rabbitmq重启的时候不需要创建新的队列 auto-delete 表示消息队列没有在使用时将被自动删除 默认是false exclusive 表示该消息队列是否只在当前connection生效,默认是false */ return new Queue("first-queue",true,false,false); } @Bean public Queue secondQueue() { return new Queue("second-queue",true,false,false); } @Bean public Queue thridQueue() { return new Queue("thrid-queue",true,false,false); } }
再创建RabbitMqConfig将指定队列和所要使用的交换机通过路由key来绑定
package com.cy.demo.rabbitmq.conf; import org.springframework.amqp.core.Binding; import org.springframework.amqp.core.BindingBuilder; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class RabbitMqConfig { /** 消息交换机的名字*/ public static final String EXCHANGE = "exchange01"; /** 队列key1*/ public static final String ROUTINGKEY1 = "queue_one_key1"; /** 队列key2*/ public static final String ROUTINGKEY2 = "queue_one_key2"; /** 队列key3*/ public static final String ROUTINGKEY3 = "queue_one_key3"; @Autowired private QueueConfig queueConfig; @Autowired private ExchangeConfig exchangeConfig; /** 将消息队列1和交换机进行绑定 */ @Bean public Binding binding_one() { return BindingBuilder.bind(queueConfig.firstQueue()).to(exchangeConfig.directExchange()).with(RabbitMqConfig.ROUTINGKEY1); } /** * 将消息队列2和交换机进行绑定 */ @Bean public Binding binding_two() { return BindingBuilder.bind(queueConfig.secondQueue()).to(exchangeConfig.directExchange()).with(RabbitMqConfig.ROUTINGKEY2); } /** * 将消息队列3和交换机进行绑定 */ @Bean public Binding binding_three() { return BindingBuilder.bind(queueConfig.thridQueue()).to(exchangeConfig.directExchange()).with(RabbitMqConfig.ROUTINGKEY3); } }
完成配置类的创建之后,就可以通过api对队列进行操作了
创建TestController简单的对队列进行操作
package com.cy.demo.rabbitmq.controller; import org.springframework.amqp.core.AmqpTemplate; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; @RestController public class TestController { //注入AmqpTemplate @Autowired private AmqpTemplate amqpTemplate; @RequestMapping(value = "/q1send",method = RequestMethod.GET) public void q1send(String str){ //将消息送入之前设置的first-queue,第二个参数为送入队列的内容, // 可以是对象也可以是其他类型 amqpTemplate.convertAndSend("first-queue",str); } //取出对应队列里的消息 @RequestMapping(value = "/receive",method = RequestMethod.GET) public void receive(String queueName){ Object object =amqpTemplate.receiveAndConvert(queueName); System.out.println(object.toString()); } }
通过启动类启动
访问localhost:8080/q1send?str=向征你好,
就可以送入队列first-queue里面了
访问http://localhost:15672,可以看到队列里的消息已经有一条了
再访问localhost:8080/receive?queueName=first-queue
把队列的名字传进去
就可以取出之前送入到队列里的消息了
这里只使用到一个队列,只做一个简单的例子
评价
排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2024TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

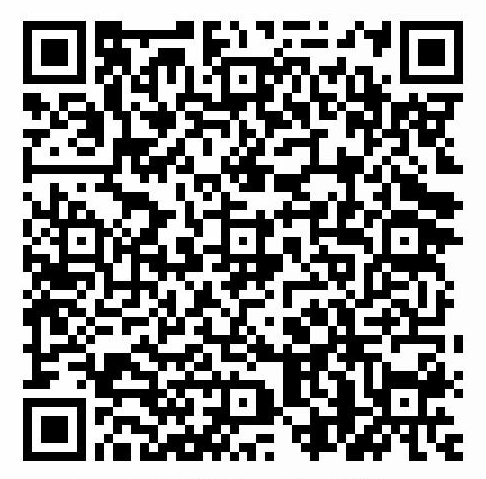
欢迎加群交流技术