
主要使用插件vue-esign
,官方文档:https://github.com/JaimeCheng/vue-esign
一:添加引用
cnpm install vue-esign
二:简单使用
先引用
import vueEsign from 'vue-esign'
就可以直接使用了
<vue-esign ref="esign" :width="800" :height="300"/>
在页面里边完整一点的引用
<template>
<div class="upLoadSign-container">
<div>
<vue-esign ref="esign" :width="800" :height="300"/>
</div>
</div>
</template>
<script setup lang="ts" name="upLoadSign">
import {reactive, onMounted} from 'vue'
import vueEsign from 'vue-esign'
const state = reactive({
title: '更新',
})
onMounted(() => {})
</script>
<style scoped="scoped" lang="scss">
.upLoadSign-container {
padding: 15px;
}
</style>
三:丰富一点的用法
包含背景颜色,线条颜色,重置的时候保留背景颜色的设置等
<template>
<div class="upLoadSign-container">
<div>线条颜色:<input type="color" v-model="lineColor" /> 背景颜色:<input type="color" v-model="bgColor" /></div>
<div class="ulsc-signContent">
<vue-esign ref="esign" :isClearBgColor="isClearBgColor" :width="560" :height="300" :lineColor="lineColor" :bgColor="bgColor" />
<div class="ulsc-sc-operate">
<el-button @click="handleReset">清空</el-button>
<el-button type="primary" @click="handleGenerate">确定</el-button>
</div>
<img :src="resultImg" />
</div>
</div>
</template>
<script setup lang="ts" name="upLoadSign">
import { reactive, onMounted, ref } from 'vue'
import vueEsign from 'vue-esign'
const bgColor = ref('#E6E0E0') //背景颜色
const lineColor = ref('') //线条颜色
const resultImg = ref('') //拿到的图片的base64
const isCrop = ref(false) //是否可裁剪
const esign = ref() //组件dom
const isClearBgColor = ref(false) //是否在重置的时候清空背景颜色
const state = reactive({
title: '更新'
})
// 清空组件
function handleReset() {
esign.value.reset()
resultImg.value = ''
}
//生成图片,并下载图片
function handleGenerate() {
esign.value
.generate()
.then((res: any) => {
resultImg.value = res
})
.catch((err: any) => {
alert(err) // 画布没有签字时会执行这里 'Not Signned'
})
}
onMounted(() => {})
</script>
<style scoped="scoped" lang="scss">
.upLoadSign-container {
padding: 15px;
.ulsc-signContent {
margin-top: 10px;
.ulsc-sc-operate{
margin-top: 10px;
display: flex;
justify-content: flex-end;
}
}
}
</style>
四:配合base64 图片上传
<template>
<div class="upLoadSign-container">
<div>线条颜色:<input type="color" v-model="lineColor" /> 背景颜色:<input type="color" v-model="bgColor" /></div>
<div class="ulsc-signContent">
<vue-esign ref="esign" :isClearBgColor="isClearBgColor" :width="560" :height="300" :lineColor="lineColor" :bgColor="bgColor" />
<div class="ulsc-sc-operate">
<el-button @click="handleReset">清空</el-button>
<el-button type="primary" @click="handleGenerate">确定</el-button>
</div>
<!-- <img :src="resultImg" /> -->
</div>
</div>
</template>
<script setup lang="ts" name="upLoadSign">
import { reactive, onMounted, ref } from 'vue'
import vueEsign from 'vue-esign'
import request from '/@/utils/requestTools'
import { getToken } from '/@/utils/auth'
const bgColor = ref('#E6E0E0') //背景颜色
const lineColor = ref('') //线条颜色
// const resultImg = ref('') //拿到的图片的base64
const isCrop = ref(false) //是否可裁剪
const esign = ref() //组件dom
const isClearBgColor = ref(false) //是否在重置的时候清空背景颜色
const state = reactive({
title: '更新'
})
// 清空组件
function handleReset() {
esign.value.reset()
// resultImg.value = ''
}
//生成图片,并下载图片
function handleGenerate() {
esign.value
.generate()
.then((res: any) => {
// resultImg.value = res
base64ToImg(res)
})
.catch((err: any) => {
alert(err) // 画布没有签字时会执行这里 'Not Signned'
})
}
// Base64 编码的图片转成二进制图片
const base64ToImg = (base64String: string) => {
const byteString = window.atob(base64String.split(',')[1])
const mimeString = base64String.split(',')[0].split(':')[1].split(';')[0]
const ab = new ArrayBuffer(byteString.length)
const ia = new Uint8Array(ab)
for (let i = 0; i < byteString.length; i++) {
ia[i] = byteString.charCodeAt(i)
}
const blob = new Blob([ab], { type: mimeString })
upLoadImg(blob)
}
// 调用图片文件上传的接口
const upLoadImg = async (blob: any) => {
const formData = new FormData()
formData.append('file', blob, 'image.png')
// 其他参数
formData.append('bucketName', "teacher-certification")
formData.append('filePath', "clients")
formData.append('fileType', "1")
fetch('/oss/api/xxFiles/UpLoadFormFile', {
method: 'POST',
headers: {
// 'Content-Type': 'application/json',
Authorization:'Bearer '+ getToken() // 替换为你的 token
},
body: formData
})
.then((response) => {
console.log('上传成功', response)
// 后面的其他业务逻辑需要自己操作
})
.catch((error) => {
console.error('上传失败', error)
})
}
onMounted(() => {})
</script>
<style scoped="scoped" lang="scss">
.upLoadSign-container {
padding: 15px;
.ulsc-signContent {
margin-top: 10px;
.ulsc-sc-operate {
margin-top: 10px;
display: flex;
justify-content: flex-end;
}
}
}
</style>
注意:fetch 获取返回值还要写一个then里边使用tojson才行
fetch 获取返回值还要写一个then里边使用tojson才行
fetch('https://api.example.com/data')
// 这里要多加一个then才能获取到返回值
.then(response => {
if (response.ok) {
return response.json(); // 转换为JSON
}
throw new Error('Network response was not ok.');
})
.then(data => console.log(data)) // 这里的data是通过JSON转换的对象
.catch(error => console.error('There has been a problem with your fetch operation:', error)); // 捕获错误
欢迎加群讨论技术,1群:677373950(满了,可以加,但通过不了),2群:656732739。有需要软件开发,或者学习软件技术的朋友可以和我联系~(Q:815170684)
评价
排名
8
文章
222
粉丝
7
评论
7
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

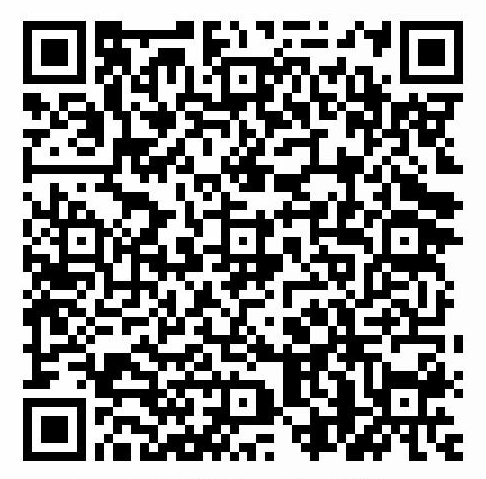
欢迎加群交流技术