
方法一:分成两份来展示,一份显示出来,一份隐藏掉,点击加载全部在把隐藏的显示出来
封装的组件的代码如下:
<template>
<div class="completeUerList-container">
<div class="cu-ct-title">
{{ props.title }}
</div>
<div class="cu-ct-users">
<div class="cu-ct-us-item" v-for="(item, index) in state.firstUsers" :key="index">
<img class="cu-ct-us-head" src="https://image.tnblog.net/dbc68332d521436c983fbc3adc6d9c7f.jpeg" />
<div class="cu-ct-us-name">
成都标榜:闵杨
<!-- <div class="cu-ct-us-spline"></div> -->
</div>
</div>
<div class="cu-ct-us-item" v-show="state.isShowMore" v-for="(item, index) in state.remainingUsers" :key="index">
<img class="cu-ct-us-head" src="https://image.tnblog.net/dbc68332d521436c983fbc3adc6d9c7f.jpeg" />
<div class="cu-ct-us-name">成都标榜:闵杨</div>
</div>
</div>
<div class="cu-ct-more" v-if="completeuCount > 10 && !state.isShowMore">
<div class="cuct-wrap" @click="loadMore">
加载全部<el-icon size="16"><ArrowDownBold class="cuct-wrap-icon" /></el-icon>
</div>
</div>
</div>
</template>
<script setup lang="ts" name="CompleteUerList">
import { defineAsyncComponent, reactive, onMounted, toRefs, ref } from 'vue'
import { ArrowDownBold } from '@element-plus/icons-vue'
const props = defineProps({
title: {
type: String,
default: '已完成任务'
},
completeUsers: {
type: [] as any,
default: []
}
})
const state = reactive({
firstUsers: [],
remainingUsers: [],
isShowMore: false
})
const completeuCount = ref(0)
onMounted(() => {
completeuCount.value = props.completeUsers.length
if (completeuCount.value > 10) {
// 分成两份来展示,默认显示10条
state.firstUsers = props.completeUsers.slice(0, 10)
state.remainingUsers = props.completeUsers.slice(10)
}
else{
state.firstUsers = props.completeUsers
}
})
// 加载更多,让另外一份隐藏的显示出来
const loadMore = () => {
state.isShowMore = true
}
</script>
<style scoped="scoped" lang="scss">
.completeUerList-container {
.cu-ct-title {
font-family: Microsoft YaHei;
font-weight: 600;
font-size: 16px;
color: #292f3d;
}
.cu-ct-users {
margin-top: 15px;
.cu-ct-us-item:last-child {
border-bottom: none;
padding-bottom: 0px;
}
.cu-ct-us-item {
display: flex;
align-items: center;
margin-bottom: 10px;
border-bottom: solid 1px #f7f7f7;
padding-bottom: 10px;
cursor: pointer;
.cu-ct-us-head {
width: 28px;
height: 28px;
border-radius: 50%;
}
.cu-ct-us-name {
font-family: Microsoft YaHei;
font-weight: 400;
font-size: 14px;
color: #60646e;
line-height: 26px;
margin-left: 15px;
}
.cu-ct-us-spline {
height: 1px;
background: #f7f7f7;
width: 300px;
margin-top: 10px;
}
}
}
.cu-ct-more {
margin-top: 13px;
margin-bottom: 7px;
text-align: center;
font-family: Microsoft YaHei;
font-weight: 400;
font-size: 14px;
color: #1880ff;
.cuct-wrap {
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
.cuct-wrap-icon {
margin-left: 5px;
}
}
}
}
</style>
对这个组件的使用:
<template>
<div class="teacher-training-container">
<CompleteUerList title="已完成任务" :completeUsers=state.completeUsers></CompleteUerList>
</div>
</template>
<script setup lang="ts" name="teacher-training-details">
const CompleteUerList = defineAsyncComponent(() => import('/@/components/CompleteUerList.vue'))
const state = reactive({
// 模拟提供数据源
completeUsers:function(){
let users = []
for (let index = 0; index < 16; index++) {
users.push({name:index})
}
return users
}(),
})
</script>
增加了一个是否显示全部的配置IsShowAll
<template>
<div class="completeUerList-container">
<div class="cu-ct-title">
{{ props.title }}
</div>
<div class="cu-ct-users">
<div class="cu-ct-us-item" v-for="(item, index) in state.firstUsers" :key="index">
<img class="cu-ct-us-head" src="https://image.tnblog.net/dbc68332d521436c983fbc3adc6d9c7f.jpeg" />
<div class="cu-ct-us-name">
成都标榜:闵杨
</div>
</div>
<div class="cu-ct-us-item" v-show="state.isShowMore" v-for="(item, index) in state.remainingUsers" :key="index">
<img class="cu-ct-us-head" src="https://image.tnblog.net/dbc68332d521436c983fbc3adc6d9c7f.jpeg" />
<div class="cu-ct-us-name">成都标榜:闵杨</div>
</div>
</div>
<div class="cu-ct-more" v-if="completeuCount > 10 &&!IsShowAll && !state.isShowMore">
<div class="cuct-wrap" @click="loadMore">
加载全部<el-icon size="16"><ArrowDownBold class="cuct-wrap-icon" /></el-icon>
</div>
</div>
</div>
</template>
<script setup lang="ts" name="CompleteUerList">
import { defineAsyncComponent, reactive, onMounted, toRefs, ref } from 'vue'
import { ArrowDownBold } from '@element-plus/icons-vue'
const props = defineProps({
title: {
type: String,
default: '已完成任务'
},
IsShowAll: {
type: Boolean,
default: false
},
completeUsers: {
type: [] as any,
default: []
}
})
const state = reactive({
firstUsers: [],
remainingUsers: [],
isShowMore: false
})
const completeuCount = ref(0)
onMounted(() => {
completeuCount.value = props.completeUsers.length
if (completeuCount.value > 10 && props.IsShowAll===false) {
state.firstUsers = props.completeUsers.slice(0, 10)
state.remainingUsers = props.completeUsers.slice(10)
}
else{
state.firstUsers = props.completeUsers
}
})
const loadMore = () => {
state.isShowMore = true
}
</script>
<style scoped="scoped" lang="scss">
.completeUerList-container {
.cu-ct-title {
font-family: Microsoft YaHei;
font-weight: 600;
font-size: 16px;
color: #292f3d;
}
.cu-ct-users {
margin-top: 15px;
.cu-ct-us-item:last-child {
border-bottom: none;
padding-bottom: 0px;
}
.cu-ct-us-item {
display: flex;
align-items: center;
margin-bottom: 10px;
border-bottom: solid 1px #f7f7f7;
padding-bottom: 10px;
cursor: pointer;
.cu-ct-us-head {
width: 28px;
height: 28px;
border-radius: 50%;
}
.cu-ct-us-name {
font-family: Microsoft YaHei;
font-weight: 400;
font-size: 14px;
color: #60646e;
line-height: 26px;
margin-left: 15px;
}
.cu-ct-us-spline {
height: 1px;
background: #f7f7f7;
width: 300px;
margin-top: 10px;
}
}
}
.cu-ct-more {
margin-top: 13px;
margin-bottom: 7px;
text-align: center;
font-family: Microsoft YaHei;
font-weight: 400;
font-size: 14px;
color: #1880ff;
.cuct-wrap {
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
.cuct-wrap-icon {
margin-left: 5px;
}
}
}
}
</style>
使用
<CompleteUerList title="已完成任务" :IsShowAll="false" :completeUsers=state.completeUsers></CompleteUerList>
欢迎加群讨论技术,1群:677373950(满了,可以加,但通过不了),2群:656732739。有需要软件开发,或者学习软件技术的朋友可以和我联系~(Q:815170684)
评价
排名
8
文章
222
粉丝
7
评论
7
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

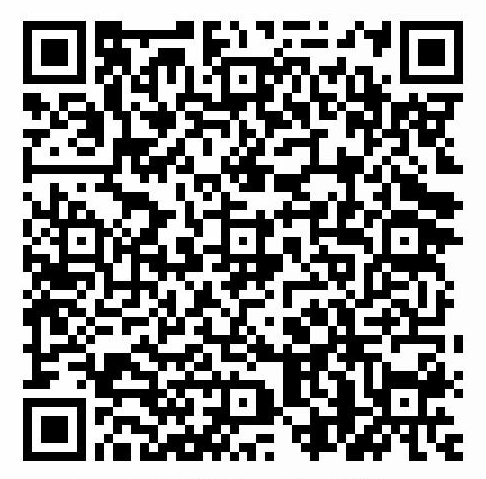
欢迎加群交流技术