排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

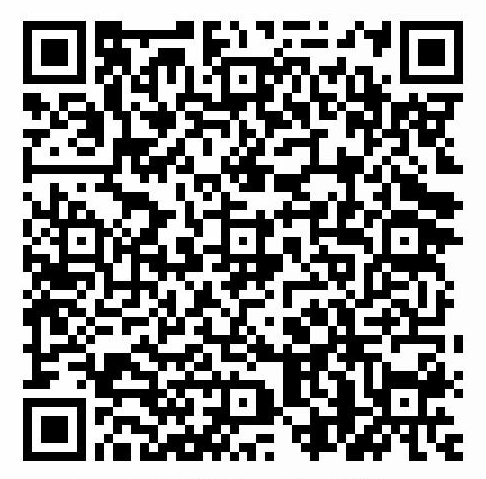
欢迎加群交流技术

下边的代码,创建控制台应用后直接Copy就OK,替换apiKey
using System; using System.Net.Http; using System.Text; using System.Threading.Tasks; using Newtonsoft.Json; using System.IO; using System.Threading; using System.Net.Http.Headers; class Program { private static readonly string apiKey = "apiKey"; private static readonly string apiUrl = "https://api.deepseek.com/v1/chat/completions"; static async Task Main(string[] args) { while (true) { Console.WriteLine("请输入您的问题:"); var question = Console.ReadLine(); if (question.ToLower() == "exit") { break; } if (string.IsNullOrEmpty(question.Trim())) { continue; } await SendToDeepSeek(question); Console.WriteLine("\n\n"); } } static async Task SendToDeepSeek(string question) { var requestData = new { model = "deepseek-chat", messages = new[] { new { role = "user", content = question } }, stream = true, // 添加流式传输参数 max_tokens = 8192 }; try { await CallDeepSeekAPIStreaming(requestData); } catch (Exception ex) { Console.WriteLine($"\nError: {ex.Message}"); } } static async Task CallDeepSeekAPIStreaming(object requestData) { using (var client = new HttpClient()) { client.DefaultRequestHeaders.Add("Authorization", $"Bearer {apiKey}"); client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json")); var jsonContent = JsonConvert.SerializeObject(requestData); var httpContent = new StringContent(jsonContent, Encoding.UTF8, "application/json"); using (var request = new HttpRequestMessage(HttpMethod.Post, apiUrl) { Content = httpContent }) using (var response = await client.SendAsync(request, HttpCompletionOption.ResponseHeadersRead)) using (var stream = await response.Content.ReadAsStreamAsync()) using (var reader = new StreamReader(stream)) { while (!reader.EndOfStream) { var line = await reader.ReadLineAsync(); if (string.IsNullOrWhiteSpace(line)) continue; // 处理事件流格式 if (line.StartsWith("data: ")) { var jsonData = line.Substring(6).Trim(); if (jsonData == "[DONE]") break; var chunk = JsonConvert.DeserializeObject<StreamChatResponse>(jsonData); var content = chunk?.choices?.FirstOrDefault()?.delta?.content; if (!string.IsNullOrEmpty(content)) { Console.Write(content); // 若需要模拟逐字效果,可添加延迟 await Task.Delay(50); } } } } } } public class StreamChatResponse { public Choice[] choices { get; set; } public class Choice { public Delta delta { get; set; } } public class Delta { public string content { get; set; } } } }
评价