
main.c
#include <stdio.h> #include <stdlib.h> #include <string.h> #include "link_list.h" #define my_malloc malloc #define my_free free //定义一个形状 struct rect { int x; int y; int m; int h; }; struct circle { int x; int y; }; struct shape { int type; union { struct rect r; struct circle c; }u; struct link_node link; }; //创建一个空间 static struct shape* alloc_shape() { struct shape* one = my_malloc(sizeof(struct shape)); memset(one, 0, sizeof(struct shape)); return one; } //释放 static void free_shape(struct shape* a) { my_free(a); } //清理单链表中所有内存 static void All_shape(hhlinknode all) { if (!all) { return; } struct shape* elem = LINK_TO_ELEM(all, struct shape, link); if (all->next) { All_shape(all->next); } free_shape(elem); } int main() { hhlinknode Thead = NULL; struct shape* shapeone = alloc_shape(); shapeone->type = 1; list_insert_head(&Thead, &shapeone->link); shapeone = alloc_shape(); shapeone->type = 2; list_insert_head(&Thead, &shapeone->link); shapeone = alloc_shape(); shapeone->type = 3; list_insert_tail(&Thead, &shapeone->link); hhlinknode walk = Thead; while (walk) { //通过偏移指针获取到类型 struct shape* elem = LINK_TO_ELEM(walk, struct shape, link); //struct shape* elem = ((struct shape*)((unsigned char*)walk - (unsigned char*)(&((struct shape*)NULL)->link))); printf("%d \n", elem->type); walk = walk->next; } list_insert_remove(&Thead, &shapeone->link); All_shape(Thead); system("pause"); return 0; }
单链表:
link_list.h
#ifndef _LINK_LIST_H_ #define _LINK_LIST_H_ typedef struct link_node{ struct link_node* next; }hlinknode,*hhlinknode; typedef struct link_node *list_head; //单链表头部添加 void list_insert_head(hhlinknode* header, hhlinknode node); //单链表尾部添加 void list_insert_tail(hhlinknode* header, hhlinknode node); //单链表指定节点删除 void list_insert_remove(hhlinknode* header, hhlinknode node); //#define LINK_TO_ELEM(link,elem_type,mem_name) (((elem_type)*)(((unsigned char*)link)-(unsigned char*)(&((elem_type*)NULL)->mem_name))) //通过指针 #define LINK_TO_ELEM(link,elem_type,mem_name) \ ((elem_type*)((unsigned char*)link - (unsigned char*)(&((elem_type*)NULL)->mem_name))); #endif
link_list.c
#include <stdio.h> #include <stdlib.h> #include <string.h> #include "link_list.h" void list_insert_head(hhlinknode* header, hhlinknode node) { hhlinknode* walk = header; node->next = *walk; *walk = node; } void list_insert_tail(hhlinknode* header, hhlinknode node) { hhlinknode* walk = header; while (*walk) { walk = &((*walk)->next); } node->next = NULL; *walk = node; } void list_insert_remove(hhlinknode* header, hhlinknode node) { hhlinknode* walk = header; while (*walk) { if (*walk==node) { *walk = node->next; node = NULL; return; } //这里必须要改指针的指针,不是改指针的值 walk = &(*walk)->next; } }
欢迎加群讨论技术,1群:677373950(满了,可以加,但通过不了),2群:656732739
评价
排名
6
文章
6
粉丝
16
评论
8
{{item.articleTitle}}
{{item.blogName}} : {{item.content}}
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2024TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

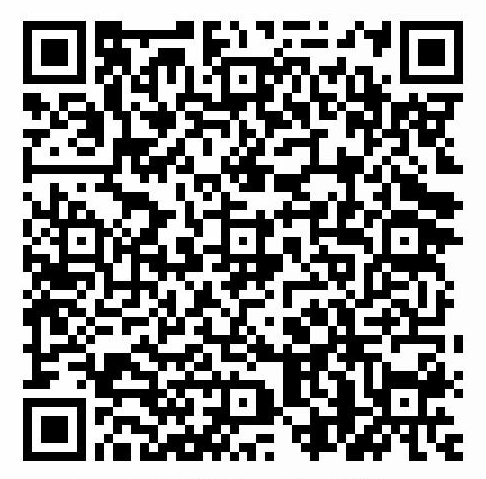
欢迎加群交流技术