
Three js 绘制星空
package.json
{
"name": "01-three_basic",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"dev": "parcel src/index.html",
"build": "parcel build src/index.html"
},
"author": "",
"license": "ISC",
"devDependencies": {
"parcel-bundler": "^1.12.5"
},
"dependencies": {
"dat.gui": "^0.7.9",
"gsap": "^3.11.5",
"three": "^0.152.2"
}
}
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="./assets/css/style.css">
</head>
<body>
<script src="./main/main.js" type="module"></script>
</body>
</html>
main.js
import * as THREE from 'three';
// 导入轨道控制器
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls'
// 导入动画库
// import { gsap } from 'gsap';
// 导入dat.gui
// import * as dat from 'dat.gui'
// 创建平面
// 创建场景
const scene = new THREE.Scene()
// 创建相机
// PerspectiveCamera 透视相机
const camera = new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 0.1, 1000)
// 设置位置
// x,y,z
camera.position.set(0, 0, 40);
// 添加相机
scene.add(camera)
// 添加辅助线
// const axesHelper = new THREE.AxesHelper( 5 );
// scene.add( axesHelper );
function createPoints(url,size = 0.5){
// 创建球几何体
const sphereGeometry = new THREE.BufferGeometry();
const count = 5000;
// 设置缓冲区数组
const positions = new Float32Array(count * 3);
// 设置顶点
const colors = new Float32Array(count * 3)
for (let i = 0; i < count * 3; i++) {
positions[i] = (Math.random() - 0.5) * 100;
colors[i] = Math.random();
}
sphereGeometry.setAttribute('position',new THREE.BufferAttribute(positions,3))
sphereGeometry.setAttribute("color", new THREE.BufferAttribute(colors, 3));
// 设置点材质
const pointMaterial = new THREE.PointsMaterial()
// 设置点大小
pointMaterial.size = 0.5;
// 设置点颜色
pointMaterial.color.set(0xfff000)
// 添加受光影响
pointMaterial.sizeAttenuation = true
// 载入纹理
const textureLoader = new THREE.TextureLoader()
const texture = textureLoader.load(`./textures/particles/${url}.png`)
// 设置纹理
pointMaterial.map = texture;
pointMaterial.alphaMap = texture;
pointMaterial.transparent = true;
pointMaterial.depthWrite = false;
pointMaterial.blending = THREE.AdditiveBlending;
// 启用顶点颜色
pointMaterial.vertexColors = true;
// 创建点
const points = new THREE.Points(sphereGeometry, pointMaterial)
// 场景添加点
scene.add( points );
return points
}
const points = createPoints("zs2",1.5)
const points2 = createPoints("xh",1)
const points3 = createPoints("6",2)
// 初始化添加渲染器
const renderer = new THREE.WebGLRenderer();
// 设置渲染的尺寸大小
renderer.setSize(window.innerWidth,window.innerHeight)
// 开启场景中的阴影贴图
renderer.shadowMap.enabled = true;
renderer.useLegacyLights = true;
// 将webgl渲染的canvas内容添加到body
document.body.appendChild(renderer.domElement)
// 创建控制器
const controls = new OrbitControls(camera, renderer.domElement)
// 设置控制器阻力,让控制器更有真实效果,必须在你的动画循环里调用.update()。
controls.enableDamping = true;
// 设置时钟
const clock = new THREE.Clock();
function render() {
let time = clock.getElapsedTime();
// points.material.map.needsUpdate = true;
// texture.needsUpdate = true
// 自动旋转
points.rotation.x = time * 0.3
points2.rotation.x = time * 0.5
points2.rotation.y = time * 0.4
points3.rotation.z = time * 0.4
// 对应设置控制器阻力
controls.update();
// 渲染render
renderer.render(scene,camera);
// 渲染下一帧的时候就会调用render函数
requestAnimationFrame( render );
}
render()
// 监听画面变化,更新渲染画面
window.addEventListener("resize", () => {
// 更新摄像头
camera.aspect = window.innerWidth / window.innerHeight;
// 更新摄像机的投影矩阵
camera.updateProjectionMatrix();
// 更新渲染器
renderer.setSize(window.innerWidth, window.innerHeight);
// 设置渲染器的像素比
renderer.setPixelRatio(window.devicePixelRatio);
});
这里有个图。
欢迎加群讨论技术,1群:677373950(满了,可以加,但通过不了),2群:656732739
评价
排名
2
文章
634
粉丝
44
评论
93
docker中Sware集群与service
尘叶心繁 : 想学呀!我教你呀
一个bug让程序员走上法庭 索赔金额达400亿日元
叼着奶瓶逛酒吧 : 所以说做程序员也要懂点法律知识
.net core 塑形资源
剑轩 : 收藏收藏
映射AutoMapper
剑轩 :
好是好,这个对效率影响大不大哇,效率高不高
一个bug让程序员走上法庭 索赔金额达400亿日元
剑轩 : 有点可怕
ASP.NET Core 服务注册生命周期
剑轩 :
http://www.tnblog.net/aojiancc2/article/details/167
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

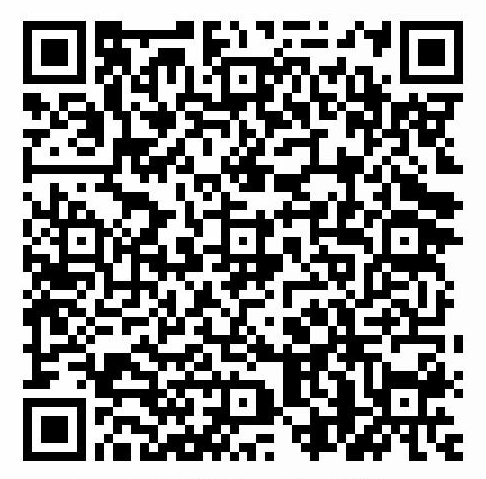
欢迎加群交流技术
小可爱
哇![[兔子]](http://www.tnblog.net/content/static/layui/images/face/51.gif)
小坑小坑